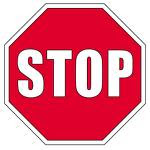
Step#1 - Reduce the resolution of the image so that it looks as clear as possible - use nearest neighbour method.
% the size of the original image is 150 by 150 pixels.
function [smallimage]=shrink2(pic,f);
Mp = floor(size(pic,1)*f);
Np = floor(size(pic,2)*f);
smallimage(:,:,1) = zeros(Mp-1,Np-1);
smallimage(:,:,2) = zeros(Mp-1,Np-1);
smallimage(:,:,3) = zeros(Mp-1,Np-1);
for i=0:(Mp-1);
for j=0:(Np-1);
a=floor((i/f)+1);
b=floor((j/f)+1);
if (a greaterthan 0)&(a lessthan (size(pic,1)))&(b greaterthan 0)&(b lessthan(size(pic,2)));
smallimage(i+1,j+1,:)=pic(a,b,:);
end;
end;
end;
endfunction;
% To show image which was scaled down by factor f = 0.2, the resulting image will be 30 by 30 pixels.
A=imread("stop.jpg");
B=shrink2(A,.2);
C=double(B)/255;
imwrite("stopstep1.png",C(:,:,1),C(:,:,2),C(:,:,3))

Step#2 - Reduce the colours of "stopstep1.png" so that there are at most 27 colours.
A=imread("stopstep1.png");
B = floor(double(A)/86)*86+42;
imwrite("stopstep2.png",double(B)(:,:,1)/255, double(B)(:,:,2)/255, double(B)(:,:,3)/255);

Step#3 - Enlarge the image using the nearest neighbour approach.
The image "stopstep2.png" will be enlarged by a factor f = 30. The resulting image "stopstep3.png" will be 900 by 900 pixels.
function [smallimage]=enlarge(pic,f);
Mp = floor(size(pic,1)*f);
Np = floor(size(pic,2)*f);
smallimage(:,:,1) = zeros(Mp-1,Np-1);
smallimage(:,:,2) = zeros(Mp-1,Np-1);
smallimage(:,:,3) = zeros(Mp-1,Np-1);
for i=0:(Mp-1);
for j=0:(Np-1);
a=floor((i/f)+1);
b=floor((j/f)+1);
if (a greaterthan 0)&(a lessthan (size(pic,1)))&(b greaterthan 0)&(b lessthan (size(pic,2)));
smallimage(i+1,j+1,:)=pic(a,b,:);
end;
end;
end;
endfunction;
A=imread("stopstep2.png");
B=enlarge(A,30);
C=double(B)/255;
imwrite("stopstep3.png",C(:,:,1),C(:,:,2),C(:,:,3))
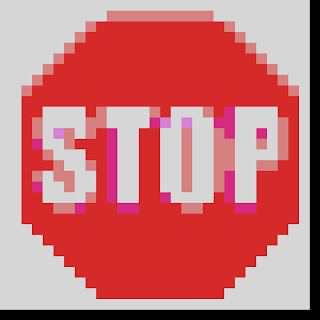
Step#4 & #5 & #6 - I will attempt to find the average colour of all visible colours within the 900 by 900 pixel image (there are 4 colours visible: red, washed-out red, pink, light pink).
%To locate a pixel and calculate its average colour, for example:.
A=imread("stopstep3.png");
B=A(240:269,60:90,:);
%To see the pixel (its colour) and calculate the average colour.
C=double(B)/255;
imwrite("stopstep4.png",C(:,:,1),C(:,:,2),C(:,:,3))
size(B)
> ans. 10 10 3
sum(sum(B))/(10*10)
> ans... (see following list of colours below)
Colourchip1 - washed out red
ans(:,:,1)=214
ans(:,:,2)=128
ans(:,:,3)=128

Colourchip2 - red
ans(:,:,1)=214
ans(:,:,2)=42
ans(:,:,3)=42

Colourchip3 - pink
ans(:,:,1)=214
ans(:,:,2)=42
ans(:,:,3)=128

Colourchip4 - light pink
ans(:,:,1)=214
ans(:,:,2)=128
ans(:,:,3)=214

Using GIMP, I adjusted the colour levels of each "stopstep1.png" image.
based on the above RGB parameters by clicking LEVELS and altering the colour levels thus, creating images that will be placed within the large image thereby creating the photomosiac. The new colour chips are now ready for placement within the large image.
Step#7 - Replace the pixels in the big image with the new smaller images which have average colours in the range {42, 128, 214} as defined in Step #2.
%Using the code from Assignment #8 Q5.
T=imread("stopstep1chip1washedred.png");
U=imread("stopstep1chip2red.png");
V=imread("stopstep1chip3pink.png");
W=imread("stopstep1chip4lightpink.png");
A=imread("stopstep3.png");
% washedred colour chips (T)
A(30:59,300:329,:)=T;
A(30:59,330:359,:)=T;
A(30:59,360:389,:)=T;
A(30:59,390:419,:)=T;
A(30:59,420:449,:)=T;
A(30:59,450:479,:)=T;
A(30:59,480:509,:)=T;
A(30:59,510:539,:)=T;
A(30:59,540:569,:)=T;
A(30:59,570:599,:)=T;
A(60:89,240:269,:)=T;
A(90:119,210:239,:)=T;
A(120:149,180:209,:)=T;
A(150:179,150:179,:)=T;
A(180:209,120:149,:)=T;
A(210:239,90:119,:)=T;
A(240:269,60:89,:)=T;
A(60:89,630:659,:)=T;
A(90:119,660:689,:)=T;
A(120:149,690:719,:)=T;
A(150:179,720:749,:)=T;
A(180:209,750:779,:)=T;
A(210:239,780:809,:)=T;
A(240:269,810:839,:)=T;
A(330:359,90:119,:)=T;
A(360:389,90:119,:)=T;
A(480:509,90:119,:)=T;
A(420:449,120:149,:)=T;
A(450:479,150:179,:)=T;
A(480:509,150:179,:)=T;
A(570:599,150:179,:)=T;
A(360:389,180:209,:)=T;
A(390:419,180:209,:)=T;
A(480:509,180:209,:)=T;
A(510:539,180:209,:)=T;
A(570:599,180:209,:)=T;
A(330:359,240:269,:)=T;
A(360:389,240:269,:)=T;
A(450:479,240:269,:)=T;
A(540:569,240:269,:)=T;
A(300:329,270:299,:)=T;
A(330:359,270:299,:)=T;
A(300:329,420:449,:)=T;
A(360:389,510:539,:)=T;
A(390:419,510:539,:)=T;
A(420:449,510:539,:)=T;
A(450:479,510:539,:)=T;
A(480:509,510:539,:)=T;
A(510:539,510:539,:)=T;
A(570:599,510:539,:)=T;
A(570:599,540:569,:)=T;
A(300:329,570:599,:)=T;
A(360:389,600:629,:)=T;
A(390:419,600:629,:)=T;
A(420:449,600:629,:)=T;
A(450:479,600:629,:)=T;
A(480:509,600:629,:)=T;
A(510:539,600:629,:)=T;
A(390:419,630:659,:)=T;
A(420:449,630:659,:)=T;
A(450:479,630:659,:)=T;
A(390:419,720:749,:)=T;
A(450:479,720:749,:)=T;
A(450:479,750:779,:)=T;
A(330:359,780:809,:)=T;
A(360:389,780:809,:)=T;
A(390:419,780:809,:)=T;
A(420:449,780:809,:)=T;
% pink colour chips (V)
A(390:419,90:119,:)=V;
A(510:539,90:119,:)=V;
A(570:599,210:239,:)=V;
A(360:389,300:329,:)=V;
A(390:419,300:329,:)=V;
A(420:449,300:329,:)=V;
A(450:479,300:329,:)=V;
A(480:509,300:329,:)=V;
A(510:539,300:329,:)=V;
A(540:569,300:329,:)=V;
A(570:599,330:359,:)=V;
A(570:599,360:389,:)=V;
A(330:359,420:449,:)=V;
A(330:359,600:629,:)=V;
A(300:329,630:659,:)=V;
A(330:359,630:659,:)=V;
A(360:389,630:659,:)=V;
A(480:509,630:659,:)=V;
A(510:539,630:659,:)=V;
A(540:569,630:659,:)=V;
A(570:599,660:689,:)=V;
A(570:599,690:719,:)=V;
A(360:389,720:749,:)=V;
A(540:569,450:479,:)=V;
% light pink colour chips
A(360:389,150:179,:)=W;
A(330:359,450:479,:)=W;
A(330:359,510:539,:)=W;
% red colour chips (U)
A(60:89,270:299,:)=U;
A(60:89,300:329,:)=U;
A(60:89,330:359,:)=U;
A(60:89,360:389,:)=U;
A(60:89,390:419,:)=U;
A(60:89,420:449,:)=U;
A(60:89,450:479,:)=U;
A(60:89,480:509,:)=U;
A(60:89,510:539,:)=U;
A(60:89,540:569,:)=U;
A(60:89,570:599,:)=U;
A(60:89,600:629,:)=U;
A(90:119,240:269,:)=U;
A(90:119,270:299,:)=U;
A(90:119,300:329,:)=U;
A(90:119,330:359,:)=U;
A(90:119,360:389,:)=U;
A(90:119,390:419,:)=U;
A(90:119,420:449,:)=U;
A(90:119,450:479,:)=U;
A(90:119,480:509,:)=U;
A(90:119,510:539,:)=U;
A(90:119,540:569,:)=U;
A(90:119,570:599,:)=U;
A(90:119,600:629,:)=U;
A(90:119,630:659,:)=U;
A(120:149,210:239,:)=U;
A(120:149,240:269,:)=U;
A(120:149,270:299,:)=U;
A(120:149,300:329,:)=U;
A(120:149,330:359,:)=U;
A(120:149,360:389,:)=U;
A(120:149,390:419,:)=U;
A(120:149,420:449,:)=U;
A(120:149,450:479,:)=U;
A(120:149,480:509,:)=U;
A(120:149,510:539,:)=U;
A(120:149,540:569,:)=U;
A(120:149,570:599,:)=U;
A(120:149,600:629,:)=U;
A(120:149,630:659,:)=U;
A(120:149,660:689,:)=U;
A(150:179,180:209,:)=U;
A(150:179,210:239,:)=U;
A(150:179,240:269,:)=U;
A(150:179,270:299,:)=U;
A(150:179,300:329,:)=U;
A(150:179,330:359,:)=U;
A(150:179,360:389,:)=U;
A(150:179,390:419,:)=U;
A(150:179,420:449,:)=U;
A(150:179,450:479,:)=U;
A(150:179,480:509,:)=U;
A(150:179,510:539,:)=U;
A(150:179,540:569,:)=U;
A(150:179,570:599,:)=U;
A(150:179,600:629,:)=U;
A(150:179,630:659,:)=U;
A(150:179,660:689,:)=U;
A(150:179,690:719,:)=U;
A(180:209,150:179,:)=U;
A(180:209,180:209,:)=U;
A(180:209,210:239,:)=U;
A(180:209,240:269,:)=U;
A(180:209,270:299,:)=U;
A(180:209,300:329,:)=U;
A(180:209,330:359,:)=U;
A(180:209,360:389,:)=U;
A(180:209,390:419,:)=U;
A(180:209,420:449,:)=U;
A(180:209,450:479,:)=U;
A(180:209,480:509,:)=U;
A(180:209,510:539,:)=U;
A(180:209,540:569,:)=U;
A(180:209,570:599,:)=U;
A(180:209,600:629,:)=U;
A(180:209,630:659,:)=U;
A(180:209,660:689,:)=U;
A(180:209,690:719,:)=U;
A(180:209,720:749,:)=U;
A(210:239,120:149,:)=U;
A(210:239,150:179,:)=U;
A(210:239,180:209,:)=U;
A(210:239,210:239,:)=U;
A(210:239,240:269,:)=U;
A(210:239,270:299,:)=U;
A(210:239,300:329,:)=U;
A(210:239,330:359,:)=U;
A(210:239,360:389,:)=U;
A(210:239,390:419,:)=U;
A(210:239,420:449,:)=U;
A(210:239,450:479,:)=U;
A(210:239,480:509,:)=U;
A(210:239,510:539,:)=U;
A(210:239,540:569,:)=U;
A(210:239,570:599,:)=U;
A(210:239,600:629,:)=U;
A(210:239,630:659,:)=U;
A(210:239,660:689,:)=U;
A(210:239,690:719,:)=U;
A(210:239,720:749,:)=U;
A(210:239,750:779,:)=U;
A(240:269,90:119,:)=U;
A(240:269,120:149,:)=U;
A(240:269,150:179,:)=U;
A(240:269,180:209,:)=U;
A(240:269,210:239,:)=U;
A(240:269,240:269,:)=U;
A(240:269,270:299,:)=U;
A(240:269,300:329,:)=U;
A(240:269,330:359,:)=U;
A(240:269,360:389,:)=U;
A(240:269,390:419,:)=U;
A(240:269,420:449,:)=U;
A(240:269,450:479,:)=U;
A(240:269,480:509,:)=U;
A(240:269,510:539,:)=U;
A(240:269,540:569,:)=U;
A(240:269,570:599,:)=U;
A(240:269,600:629,:)=U;
A(240:269,630:659,:)=U;
A(240:269,660:689,:)=U;
A(240:269,690:719,:)=U;
A(240:269,720:749,:)=U;
A(240:269,750:779,:)=U;
A(240:269,780:809,:)=U;
A(270:299,60:89,:)=U;
A(270:299,90:119,:)=U;
A(270:299,120:149,:)=U;
A(270:299,150:179,:)=U;
A(270:299,180:209,:)=U;
A(270:299,210:239,:)=U;
A(270:299,240:269,:)=U;
A(270:299,270:299,:)=U;
A(270:299,300:329,:)=U;
A(270:299,330:359,:)=U;
A(270:299,360:389,:)=U;
A(270:299,390:419,:)=U;
A(270:299,420:449,:)=U;
A(270:299,450:479,:)=U;
A(270:299,480:509,:)=U;
A(270:299,510:539,:)=U;
A(270:299,540:569,:)=U;
A(270:299,570:599,:)=U;
A(270:299,600:629,:)=U;
A(270:299,630:659,:)=U;
A(270:299,660:689,:)=U;
A(270:299,690:719,:)=U;
A(270:299,720:749,:)=U;
A(270:299,750:779,:)=U;
A(270:299,780:809,:)=U;
A(270:299,810:839,:)=U;
A(300:329,60:89,:)=U;
A(300:329,90:119,:)=U;
A(300:329,240:269,:)=U;
A(300:329,450:479,:)=U;
A(300:329,600:629,:)=U;
A(300:329,780:809,:)=U;
A(300:329,810:839,:)=U;
A(330:359,60:89,:)=U;
A(330:359,810:839,:)=U;
A(360:389,60:89,:)=U;
A(360:389,270:299,:)=U;
A(360:389,390:419,:)=U;
A(360:389,420:449,:)=U;
A(360:389,810:839,:)=U;
A(390:419,60:89,:)=U;
A(390:419,210:239,:)=U;
A(390:419,240:269,:)=U;
A(390:419,270:299,:)=U;
A(390:419,390:419,:)=U;
A(390:419,420:449,:)=U;
A(390:419,810:839,:)=U;
A(420:449,60:89,:)=U;
A(420:449,90:119,:)=U;
A(420:449,240:269,:)=U;
A(420:449,270:299,:)=U;
A(420:449,390:419,:)=U;
A(420:449,420:449,:)=U;
A(420:449,810:839,:)=U;
A(450:479,60:89,:)=U;
A(450:479,90:119,:)=U;
A(450:479,120:149,:)=U;
A(450:479,270:299,:)=U;
A(450:479,390:419,:)=U;
A(450:479,420:449,:)=U;
A(450:479,780:809,:)=U;
A(450:479,810:839,:)=U;
A(480:509,60:89,:)=U;
A(480:509,270:299,:)=U;
A(480:509,390:419,:)=U;
A(480:509,420:449,:)=U;
A(480:509,720:749,:)=U;
A(480:509,750:779,:)=U;
A(480:509,780:809,:)=U;
A(480:509,810:839,:)=U;
A(510:539,60:89,:)=U;
A(510:539,270:299,:)=U;
A(510:539,390:419,:)=U;
A(510:539,420:449,:)=U;
A(510:539,720:749,:)=U;
A(510:539,750:779,:)=U;
A(510:539,780:809,:)=U;
A(510:539,810:839,:)=U;
A(540:569,60:89,:)=U;
A(540:569,90:119,:)=U;
A(540:569,270:299,:)=U;
A(540:569,390:419,:)=U;
A(540:569,420:449,:)=U;
A(540:569,600:629,:)=U;
A(540:569,720:749,:)=U;
A(540:569,750:779,:)=U;
A(540:569,780:809,:)=U;
A(540:569,810:839,:)=U;
A(570:599,60:89,:)=U;
A(570:599,90:119,:)=U;
A(570:599,120:149,:)=U;
A(570:599,240:269,:)=U;
A(570:599,270:299,:)=U;
A(570:599,300:329,:)=U;
A(570:599,390:419,:)=U;
A(570:599,420:449,:)=U;
A(570:599,450:479,:)=U;
A(570:599,480:509,:)=U;
A(570:599,570:599,:)=U;
A(570:599,600:629,:)=U;
A(570:599,630:659,:)=U;
A(570:599,720:749,:)=U;
A(570:599,750:779,:)=U;
A(570:599,780:809,:)=U;
A(570:599,810:839,:)=U;
A(600:629,60:89,:)=U;
A(600:629,90:119,:)=U;
A(600:629,120:149,:)=U;
A(600:629,150:179,:)=U;
A(600:629,180:209,:)=U;
A(600:629,210:239,:)=U;
A(600:629,240:269,:)=U;
A(600:629,270:299,:)=U;
A(600:629,300:329,:)=U;
A(600:629,330:359,:)=U;
A(600:629,360:389,:)=U;
A(600:629,390:419,:)=U;
A(600:629,420:449,:)=U;
A(600:629,450:479,:)=U;
A(600:629,480:509,:)=U;
A(600:629,510:539,:)=U;
A(600:629,540:569,:)=U;
A(600:629,570:599,:)=U;
A(600:629,600:629,:)=U;
A(600:629,630:659,:)=U;
A(600:629,660:689,:)=U;
A(600:629,690:719,:)=U;
A(600:629,720:749,:)=U;
A(600:629,750:779,:)=U;
A(600:629,780:809,:)=U;
A(600:629,810:839,:)=U;
A(630:659,90:119,:)=U;
A(630:659,120:149,:)=U;
A(630:659,150:179,:)=U;
A(630:659,180:209,:)=U;
A(630:659,210:239,:)=U;
A(630:659,240:269,:)=U;
A(630:659,270:299,:)=U;
A(630:659,300:329,:)=U;
A(630:659,330:359,:)=U;
A(630:659,360:389,:)=U;
A(630:659,390:419,:)=U;
A(630:659,420:449,:)=U;
A(630:659,450:479,:)=U;
A(630:659,480:509,:)=U;
A(630:659,510:539,:)=U;
A(630:659,540:569,:)=U;
A(630:659,570:599,:)=U;
A(630:659,600:629,:)=U;
A(630:659,630:659,:)=U;
A(630:659,660:689,:)=U;
A(630:659,690:719,:)=U;
A(630:659,720:749,:)=U;
A(630:659,750:779,:)=U;
A(630:659,780:809,:)=U;
A(660:689,120:149,:)=U;
A(660:689,150:179,:)=U;
A(660:689,180:209,:)=U;
A(660:689,210:239,:)=U;
A(660:689,240:269,:)=U;
A(660:689,270:299,:)=U;
A(660:689,300:329,:)=U;
A(660:689,330:359,:)=U;
A(660:689,360:389,:)=U;
A(660:689,390:419,:)=U;
A(660:689,420:449,:)=U;
A(660:689,450:479,:)=U;
A(660:689,480:509,:)=U;
A(660:689,510:539,:)=U;
A(660:689,540:569,:)=U;
A(660:689,570:599,:)=U;
A(660:689,600:629,:)=U;
A(660:689,630:659,:)=U;
A(660:689,660:689,:)=U;
A(660:689,690:719,:)=U;
A(660:689,720:749,:)=U;
A(660:689,750:779,:)=U;
A(690:719,150:179,:)=U;
A(690:719,180:209,:)=U;
A(690:719,210:239,:)=U;
A(690:719,240:269,:)=U;
A(690:719,270:299,:)=U;
A(690:719,300:329,:)=U;
A(690:719,330:359,:)=U;
A(690:719,360:389,:)=U;
A(690:719,390:419,:)=U;
A(690:719,420:449,:)=U;
A(690:719,450:479,:)=U;
A(690:719,480:509,:)=U;
A(690:719,510:539,:)=U;
A(690:719,540:569,:)=U;
A(690:719,570:599,:)=U;
A(690:719,600:629,:)=U;
A(690:719,630:659,:)=U;
A(690:719,660:689,:)=U;
A(690:719,690:719,:)=U;
A(690:719,720:749,:)=U;
A(720:749,180:209,:)=U;
A(720:749,210:239,:)=U;
A(720:749,240:269,:)=U;
A(720:749,270:299,:)=U;
A(720:749,300:329,:)=U;
A(720:749,330:359,:)=U;
A(720:749,360:389,:)=U;
A(720:749,390:419,:)=U;
A(720:749,420:449,:)=U;
A(720:749,450:479,:)=U;
A(720:749,480:509,:)=U;
A(720:749,510:539,:)=U;
A(720:749,540:569,:)=U;
A(720:749,570:599,:)=U;
A(720:749,600:629,:)=U;
A(720:749,630:659,:)=U;
A(720:749,660:689,:)=U;
A(720:749,690:719,:)=U;
A(750:779,210:239,:)=U;
A(750:779,240:269,:)=U;
A(750:779,270:299,:)=U;
A(750:779,300:329,:)=U;
A(750:779,330:359,:)=U;
A(750:779,360:389,:)=U;
A(750:779,390:419,:)=U;
A(750:779,420:449,:)=U;
A(750:779,450:479,:)=U;
A(750:779,480:509,:)=U;
A(750:779,510:539,:)=U;
A(750:779,540:569,:)=U;
A(750:779,570:599,:)=U;
A(750:779,600:629,:)=U;
A(750:779,630:659,:)=U;
A(750:779,660:689,:)=U;
A(780:809,240:269,:)=U;
A(780:809,270:299,:)=U;
A(780:809,300:329,:)=U;
A(780:809,330:359,:)=U;
A(780:809,360:389,:)=U;
A(780:809,390:419,:)=U;
A(780:809,420:449,:)=U;
A(780:809,450:479,:)=U;
A(780:809,480:509,:)=U;
A(780:809,510:539,:)=U;
A(780:809,540:569,:)=U;
A(780:809,570:599,:)=U;
A(780:809,600:629,:)=U;
A(780:809,630:659,:)=U;
A(810:839,270:299,:)=U;
A(810:839,300:329,:)=U;
A(810:839,330:359,:)=U;
A(810:839,360:389,:)=U;
A(810:839,390:419,:)=U;
A(810:839,420:449,:)=U;
A(810:839,450:479,:)=U;
A(810:839,480:509,:)=U;
A(810:839,510:539,:)=U;
A(810:839,540:569,:)=U;
A(810:839,570:599,:)=U;
A(810:839,600:629,:)=U;
F=double(A)/255;
imwrite("final.png",F(:,:,1),F(:,:,2),F(:,:,3))
Original Image
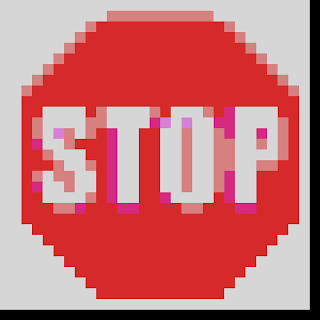
Final Image
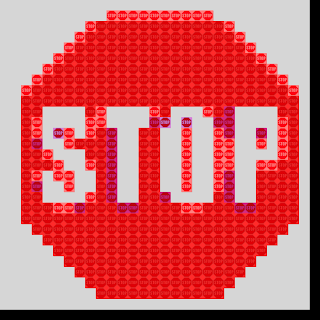
No comments:
Post a Comment