function [smallimage]=shrink2(girl,f);
Mp = floor(size(girl,1)*f);
Np = floor(size(girl,2)*f);
smallimage(:,:,1) = zeros(Mp-1,Np-1);
smallimage(:,:,2) = zeros(Mp-1,Np-1);
smallimage(:,:,3) = zeros(Mp-1,Np-1);
for i=0:(Mp-1);
for j=0:(Np-1);
a=floor((i/f)+1);
b=floor((j/f)+1);
if (a>0)&(a<(size(girl,1)))&(b>0)&(b<(size(girl,2)));
smallimage(i+1,j+1,:)=girl(a,b,:);
end;
end;
end;
endfunction;
To show the image on Octave, I used these commands:
A=imread("girl.jpg");
B=shrink2(A,f);
C=double(B)/255;
imwrite("girl75.jpg",C(:,:,1),C(:,:,2),C(:,:,3))
Using this command, you can differ the f factor and receive these scale reductions.
f=0.75 f=0.25 f=0.1
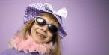


The same was done for the other two pictures.
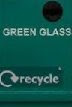


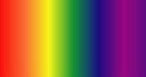


Method 3 - Bilinear Interpolation
function [smallimage]=shrink3(girl,f);
girl=double(imread("girl.jpg"));
Mp = floor(size(girl,1)*f);
Np = floor(size(girl,2)*f);
smallimage(:,:,1) = zeros(Mp-1,Np-1);
smallimage(:,:,2) = zeros(Mp-1,Np-1);
smallimage(:,:,3) = zeros(Mp-1,Np-1);
for i = 0:(Mp-1);
for j = 0:(Np-1);
a = i/f;
b = j/f;
r = floor(a);
s = floor(b);
if (r greaterthan 0)&(r lessthan256)&(s greaterthan 0)&(s lessthan256);
for k=1:3;
smallimage(i,j,k) = [1–a+r,a–r]*[girl(r,s,k), girl(r,s+1,k); girl(r+1,s,k),girl(r+1,s+1,k)]*[1–b+s;b–s];
end;
end;
end;
end;
endfunction;


The next two pics and their f factor shrink.
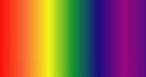


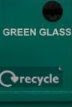


Method 1 : Average over all pixels.
function [smallimage]=shrink1(girl,f);
girl=double(imread("girl.jpg"));
Mp = floor(size(girl,1)*f);
Np = floor(size(girl,2)*f);
smallimage(:,:,1) = zeros(Mp,Np);
smallimage(:,:,2) = zeros(Mp,Np);
smallimage(:,:,3) = zeros(Mp,Np);
for i = 0 : (Mp – 1);
for j = 0 : (Np – 1);
for x =floor(i/f): ceil((i + 1)/f)-1;
for y =floor(j/f): ceil((j + 1)/f)-1;
ival=girl(x+1,y+1,:);
if (x lessthan (i/f));
ival=ival*(1+x–(i/f));
end;
if ((x + 1) > ((i + 1)/f));
ival = ival * (1 – (x + 1)+((i + 1)/f));
end;
if (y < (j/f)); ival = ival * (1 + y – (j/f)); end; if ((y + 1) > ((j + 1)/f));
ival = ival * (1 – (y + 1)+((j + 1)/f));
end;
smallimage(i+1,j+1,:) = smallimage(i+1,j+1,:) + ival;
end;
end;
smallimage(i+1,j+1,:)=smallimage(i+1,j+1,:)/(1/f)^2;
end;
end;
endfunction;
to show image:
A=imread('picturename.jpg');
B=shrink1(A,f);
C=double(B)/255;
imwrite("end.jpg",C(:,:,1),C(:,:,2),C(:,:,3))
These are the pics of "girl.jpg" with f factors 0.75, 0.25, and 0.10 respectively.
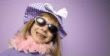


Here are the two other pics with their shrink factors using the average method.
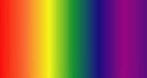


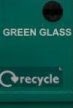


No comments:
Post a Comment