C=zeros(256);
for x = 1:256;
for y = 1:256;
if (x-100)^2+(y-100)^2<=50^2;
C(x,y) = 1 ;
endif;
endfor;
endfor;
imshow(C*255) ;
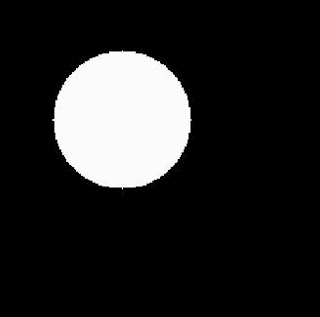
2.The commands to draw the top part of figure 6.4 in the book is:
topcircle = zeros(256);
for x = 1:256;
for y = 1:256;
if (x-90)^2 + (y-125)^2 <= 50^2;
topcircle(x, y) = 1;
endif;
endfor;
endfor;
leftcircle = zeros(256);
for x = 1:256;
for y = 1:256;
if (x-150)^2 + (y-90)^2 <= 50^2;
leftcircle(x, y) = 1;
endif;
endfor;
endfor;
rightcircle = zeros(256);
for x = 1:256;
for y = 1:256;
if (x-150)^2 + (y-150)^2 <= 50^2;
rightcircle(x, y) = 1;
endif;
endfor;
endfor;
Final(:,:,1) = topcircle;
Final(:,:,2) = leftcircle;
Final(:,:,3) = rightcircle;
imshow(Final*255);
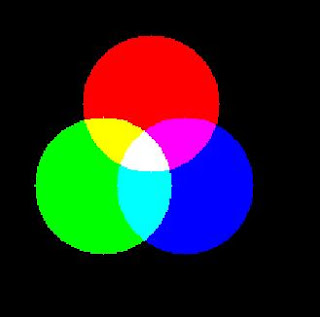
3.a) bigT=255*ones(256);
bigT(30:79,64:191)=zeros(50,128);
bigT(50:199,111:146)=zeros(150, 36);
for x = 1:256;
for y = 1:256 ;
newy=rem(2*x+y,256)+1;
bigTskew(x,y)=bigT(x,newy);
end;
end;
imshow(bigTskew);
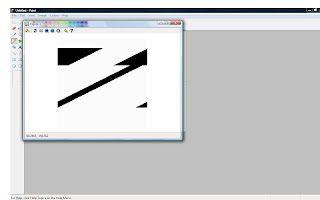
b) rotate image by 3pi/4 degrees.
bigT=255*ones(256);
bigT(30:79,64:191)=zeros(50,128);
bigT(50:199,111:146)=zeros(150, 36);
for x = 1:256
for y = 1:256
newy=rem(2*x+y,256)+1;
bigTskew(x,y)=bigT(x,newy);
end;
end;
for x=1:256;
for y=1:256;
bigTskewrotate(x,y)=bigTskew(mod(round(x*cos(3*pi/4)-y*sin(3*pi/4)),256)+1, mod(round(x*sin(3*pi/4)+y*cos(3*pi/4)),256)+1);
end;
end;
imshow(bigTskewrotate);
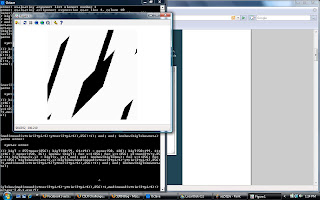